[Java] 백준 2577번
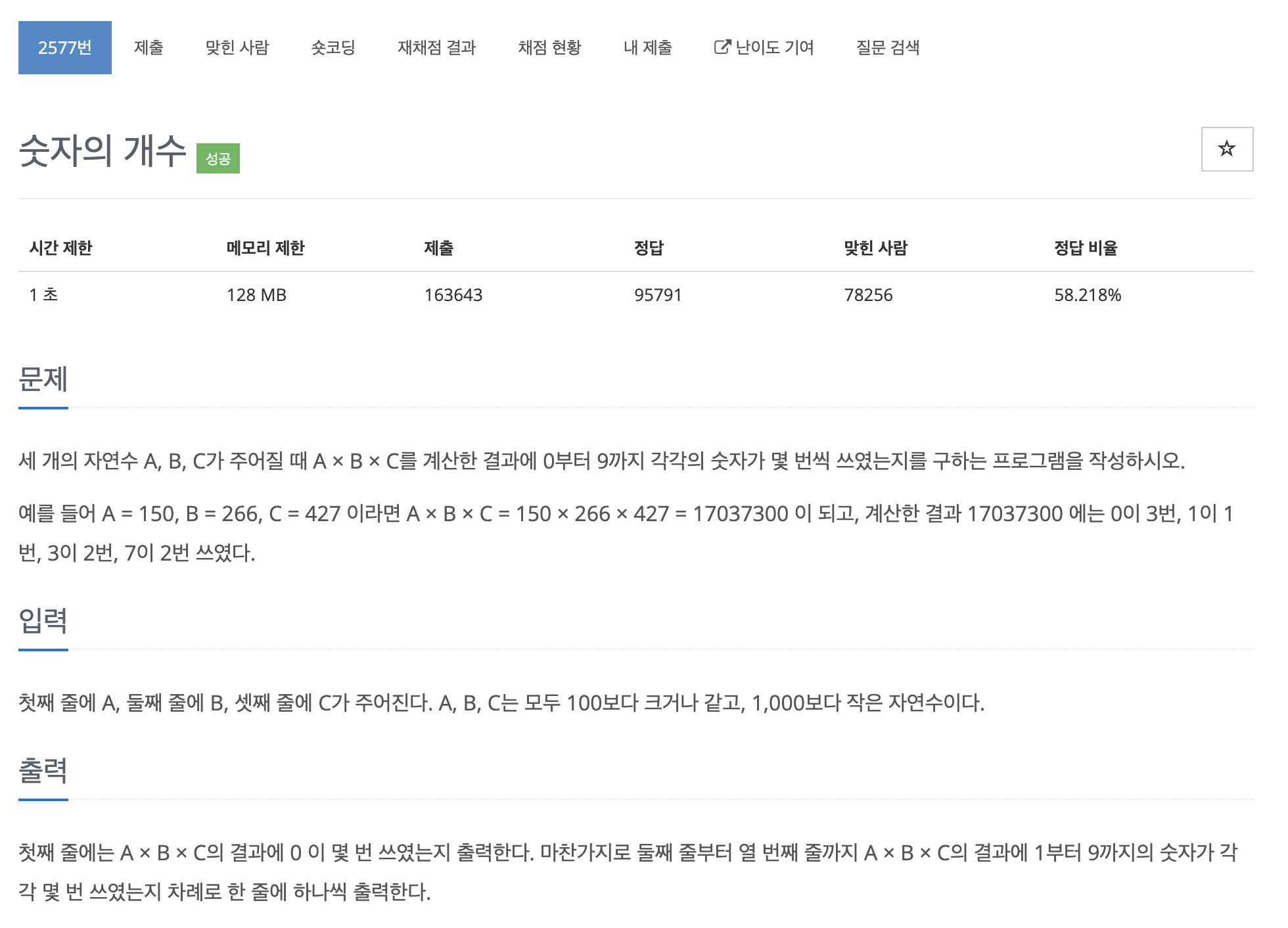
Input-1)
150
266
427
Output-1)
3
1
0
2
0
0
0
2
0
0
접근 방식
a, b, c를 곱한 값을 arr(배열)로 가지고 있다고 하면, 0부터 9까지의 인덱스가 arr의 값과 중복되는지를 검증하여 세면 될 것이라 생각했다.
먼저 a, b, c를 곱하여 arr이라는 배열로 파싱한다.
1
2
// 세 수를 곱한 값을 배열로 파싱
String[] arr = String.valueOf(a*b*c).split("");
그리고 반복문을 통해 0~9까지 돌며 arr 배열의 값과 같은지를 검증한다. 같다면 cnt(개수)를 증가시킨다. 바깥 반복문 사이클이 한바퀴씩 돌때마다 cnt를 0으로 초기화하여 인덱스별 개수를 세도록 하였다.
1
2
3
4
5
6
7
for (int i=0; i<=9; i++) {
cnt = 0; // i가 한바퀴 돌때마다 cnt 초기화
for (int j=0; j<arr.length; j++) { // arr를 돌며 같으면 cnt 증가
if(i == Integer.parseInt(arr[j])) cnt += 1;
}
counter.put(Integer.toString(i), cnt); // 현재 i까지 저장된 cnt를 counter 맵에 저장
}
작성코드
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.util.HashMap;
class Main {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
int a = Integer.parseInt(br.readLine());
int b = Integer.parseInt(br.readLine());
int c = Integer.parseInt(br.readLine());
int cnt = 0;
String[] arr = String.valueOf(a*b*c).split("");
HashMap<String, Integer> counter = new HashMap<>();
for (int i=0; i<=9; i++) {
cnt = 0;
for (int j=0; j<arr.length; j++) {
if(i == Integer.parseInt(arr[j])) cnt += 1;
}
counter.put(Integer.toString(i), cnt);
}
for (int res : counter.values()) bw.write(res + "\n");
bw.flush();
bw.close();
br.close();
}
}