[Java] 프로그래머스(level1) - 문자열 내 마음대로 정렬하기
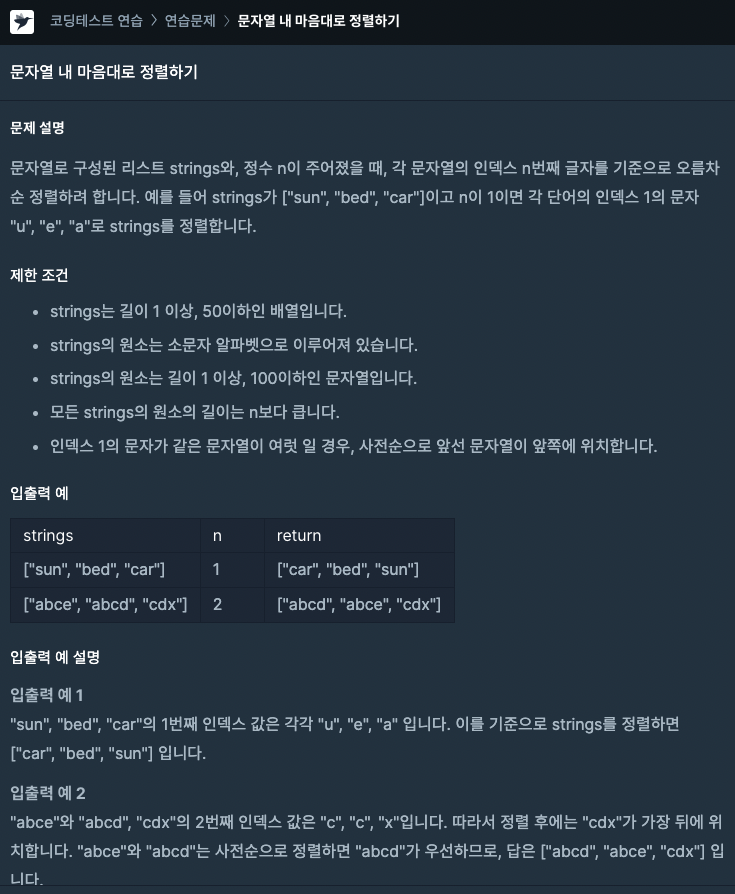
Input-1
strings = [sun, bed, car]
n = 1
Output-1
[car, bed, sun]
Input-2
strings = [abce, abcd, cdx]
n = 2
Output-2
[abcd, abce, cdx]
문제 풀이
기준 문자열의 인덱스를 순서대로 본 문자열을 정렬해야 한다.
나는 소문자 전체를 돌며 검증하는 방식으로 풀었는데 다른 분의 풀이를 보고 부족함을 많이 느꼈다..
a부터 z까지 확인하여 검증하는 방식
소문자 a부터 z까지 순회(i)하며 문자열의 n번째 인덱스를 찾아 answer 배열에 넣는다.
a부터 z까지 정렬된 순서로 반복하기에 사전순으로 검증할 수 있다.
1
2
3
4
5
for(int i=97; i<123; i++) {
for(int j=0; j<strings.length; j++) {
if((char)i == strings[j].charAt(n)) answer[idx++] = strings[j];
}
}
그런데 내가 푼 방식보다 더 좋은 풀이를 보았다..
한번 알아보자.
기준 문자열을 붙이는 방식
1
2
3
4
5
6
7
for(int i=0; i<strings.length; i++) { // 1
answer[i] = strings[i].charAt(n) + strings[i];
}
Arrays.sort(answer); // 2
for(int i=0; i<strings.length; i++) { // 3
answer[i] = answer[i].substring(1);
}
코드를 분석하기 위해 예시를 들어 생각해보자.
1
2
3
4
strings = [abce, abcd, cdx], n = 2
1. answer = [cabce, cabcd, xcdx]
2. answer = [cabcd, cabce, xcdx]
3. answer = [abcd, abce, cdx]
abce와 abcd의 기준 문자열이 c로 같을 때 c를 앞에 붙이고 정렬하면 사전순으로 정렬이 가능하다.
그렇게 깔끔하게 기준되는 문자열의 인덱스 순서로 정렬할 수 있다.
작성 코드
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
import java.util.*;
class Solution {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String[] s = sc.nextLine().split(" ");
int n = sc.nextInt();
long start = System.currentTimeMillis();
solution1(s, n);
long end = System.currentTimeMillis();
System.out.println("수행시간 = " + (end-start));
}
// a부터 z까지 확인하여 검증하는 방식
public static String[] solution1(String[] strings, int n) {
String[] answer = new String[strings.length];
int idx = 0;
Arrays.sort(strings);
for(int i=97; i<123; i++) {
for(int j=0; j<strings.length; j++) {
if((char)i == strings[j].charAt(n)) answer[idx++] = strings[j];
}
}
return answer;
}
// 기준 문자열을 붙이는 방식
public static String[] solution2(String[] strings, int n) {
String[] answer = new String[strings.length];
for(int i=0; i<strings.length; i++) {
answer[i] = strings[i].charAt(n) + strings[i];
}
Arrays.sort(answer);
for(int i=0; i<strings.length; i++) {
answer[i] = answer[i].substring(1);
}
return answer;
}
}
회고
- char형의 알파벳으로만 접근하려고 하니 다른 관점에서 전혀 생각해 볼 수 없었다. 다른 분들의 풀이를 보며 여러 관점에서 문제에 접근할 수 있음을 깨달았고 많이 배웠다.
- 기준 문자열을 본 문자열에 붙여 정렬하면 사전순으로 정렬된다는 사실에 감탄을 금치 못했다.