[Java] 프로그래머스(level-2) - 이진 변환 반복하기
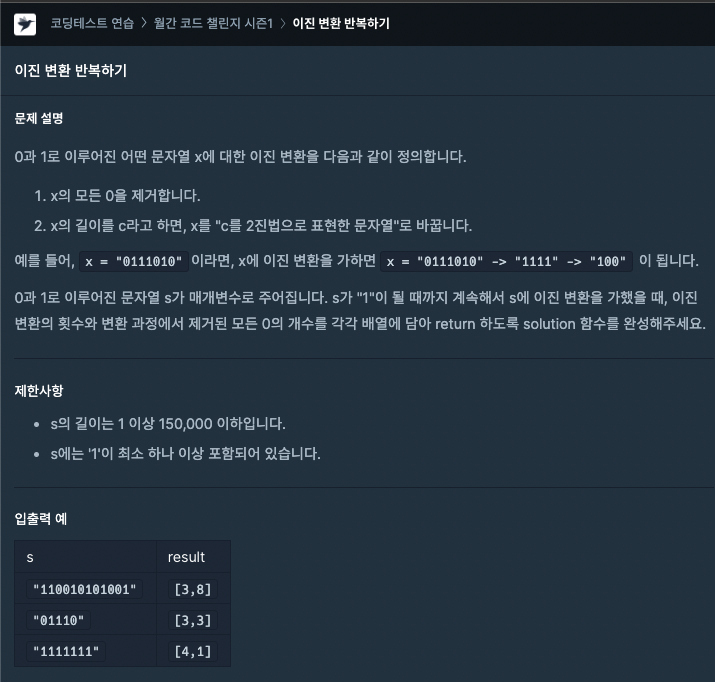
입출력 예시
Input-1
“110010101001”
Output-1
[3, 8]
Input-2
“01110”
Output-2
[3, 3]
Input-3
“1111111”
Output-3
[4, 1]
문제 풀이
주어진 문자열이 1이 될 때까지 이진 변환을 반복해야 하기 때문에 재귀함수를 활용하여 풀어보았다.
재귀함수에서 진행해야할 이진 변환 내용을 코드로 작성해보자.
문자열의 모든 0을 제거하기
1
2
3
4
5
StringBuilder sb = new StringBuilder();
for(int i=0; i<s.length(); i++) {
if(s.charAt(i) == '0') zero++;
else sb.append(s.charAt(i));
}
문자열에서 0이 아닐 경우에만 StringBuilder에 담아두었다.
문자열 길이를 2진법으로 표현하기
1
s = Integer.toBinaryString(sb.length());
Integer.toBunartString() 메서드를 활용해 0을 제거한 문자열의 길이를 2진수로 변환하였다.
1
cnt++;
위와 같은 이진변환이 한 번 이루어질 때마다 이진변환 횟수 cnt를 증가시켰다.
이진변환 이후 문자열 검증
1
2
if(s.equals("1")) return new int[]{Integer.parseInt(s), cnt, zero};
else return BinaryConversion(s, cnt, zero);
이진변환되어 문자열 s가 1이 된다면 그때의 이진변환 횟수와 제거한 0의 개수를 반환하고,
1이 아니라면, 다시 재귀함수를 통해 이진변환을 진행하도록 작성하였다.
작성 코드
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
import java.util.*;
class Solution {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String s = sc.nextLine();
long start = System.currentTimeMillis();
solution(s);
long end = System.currentTimeMillis();
System.out.println("\n수행시간 = " + (end-start));
}
public static int[] solution(String s) {
int cnt = 0;
int zero = 0;
int[] tmp = BinaryConversion(s, cnt, zero);
return new int[]{cnt, zero};
}
public static int[] BinaryConversion(String s, int cnt, int zero) {
StringBuilder sb = new StringBuilder();
for(int i=0; i<s.length(); i++) {
if(s.charAt(i) == '0') zero++;
else sb.append(s.charAt(i));
}
s = Integer.toBinaryString(sb.length());
cnt++;
if(s.equals("1")) return new int[]{Integer.parseInt(s), cnt, zero};
else return BinaryConversion(s, cnt, zero);
}
}
회고
- 문제 요구사항을 보고 재귀함수를 활용해 접근할 수 있을 것이라 생각했다.