[Java] 프로그래머스(level-2) - 다음 큰 숫자
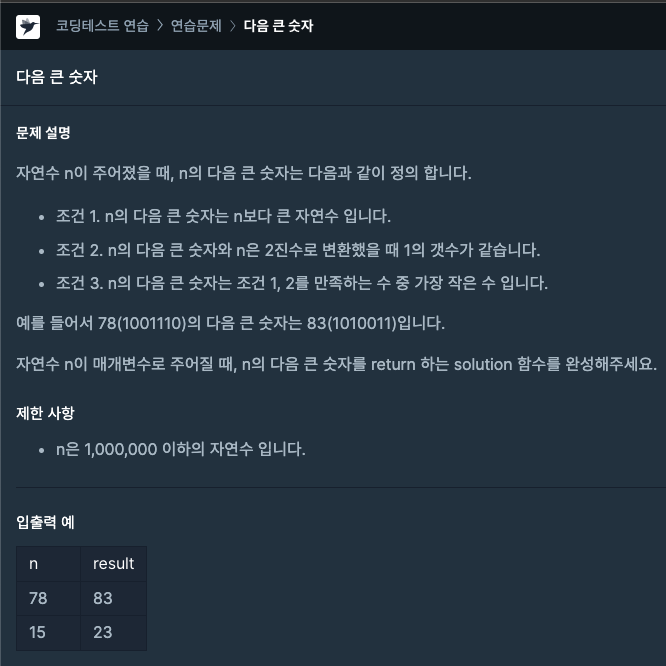
입출력 예시
Input-1
78
Output-1
83
Input-2
15
Output-2
23
문제 풀이
주어진 n을 2진수로 변환한 후 1의 개수를 cnt라고 하면
n+1, n+2 ••• n+n n보다 큰 수 중 2진수로 변환한 후의 1의 개수가 cnt와 같은 수를 구해야 하며, n보다 크면서 가장 작은 수여야 한다.
백문이 불여일견, 한 번 코드로 작성해보자.
입력 숫자 n을 2진수로 변환하고 2진수 n을 순회하며 1일 때의 카운트를 cnt에 담는다.
1
2
3
4
5
String first = Integer.toBinaryString(n);
int cnt = 0;
for(int i=0; i<first.length(); i++) {
if(first.charAt(i) == '1') cnt++;
}
그리고 n을 1씩 증가시키면서 2진수로 변환한 후 1의 개수가 cnt와 같은지를 비교한다.
1
2
3
4
5
6
int idx = n;
int nextCnt = 0;
while(nextCnt!=cnt) {
idx++;
nextCnt = nextOneCount(idx);
}
n보다 큰 수를 2진수로 변환한 후 1의 개수를 세는 nextOneCount 함수를 작성하였다.
1
2
3
4
5
6
7
8
public static int nextOneCount(int num) {
int cnt = 0;
String numberToBinaryString = Integer.toBinaryString(num);
for(int i=0; i<numberToBinaryString.length(); i++) {
if(numberToBinaryString.charAt(i) == '1') cnt++;
}
return cnt;
}
Integer.bitCount() 메서드 활용
위에서 작성한 코드도 모든 테스트케이스를 통과했지만, 코드를 간결하고 가독성이 좋도록 작성할 수 없을까 고민하던중 Integer 클래스의 bitCount() 메서드를 알게되었다.
bitCount(int n) 메서드란?
주어진 n을 2진수로 변환한 후 1의 개수를 구해준다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
public static int solution(int n) {
String first = Integer.toBinaryString(n);
int cnt = Integer.bitCount(n);
int idx = n;
int nextCnt = 0;
while(nextCnt!=cnt) {
idx++;
nextCnt = nextOneCount2(idx);
}
return idx;
}
public static int nextOneCount2(int num) {
return Integer.bitCount(num);
}
n을 2진수로 변환한 후 1의 개수를 세는 cnt를 초기화할 때, nextOneCount() 함수에서 n보다 큰 수를 2진수로 변환하여 1의 개수를 셀 때
bitCount() 메서드를 활용하여 푸니 더 간결한 코드를 작성할 수 있었다.
bitCount() 메서드를 접목한 코드도 제출하니 모든 테스트케이스와 효율성 테스트를 통과하였다.
작성 코드
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
import java.util.*;
class Solution {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
long start = System.currentTimeMillis();
solution(n);
long end = System.currentTimeMillis();
System.out.println("\n수행시간 = " + (end-start));
}
public static int solution(int n) {
String first = Integer.toBinaryString(n);
int cnt = Integer.bitCount(n);
int idx = n;
int nextCnt = 0;
while(nextCnt!=cnt) {
idx++;
nextCnt = nextOneCount2(idx);
}
return idx;
}
public static int nextOneCount(int num) {
int cnt = 0;
String numberToBinaryString = Integer.toBinaryString(num);
for(int i=0; i<numberToBinaryString.length(); i++) {
if(numberToBinaryString.charAt(i) == '1') cnt++;
}
return cnt;
}
public static int nextOneCount2(int num) {
return Integer.bitCount(num);
}
}
회고
- 2진수로 변환한 후 1의 개수를 구해주는 Integer 클래스의 bitCount() 메서드에 대해 공부할 수 있었다.