[Java] 프로그래머스(level-2) - H-index
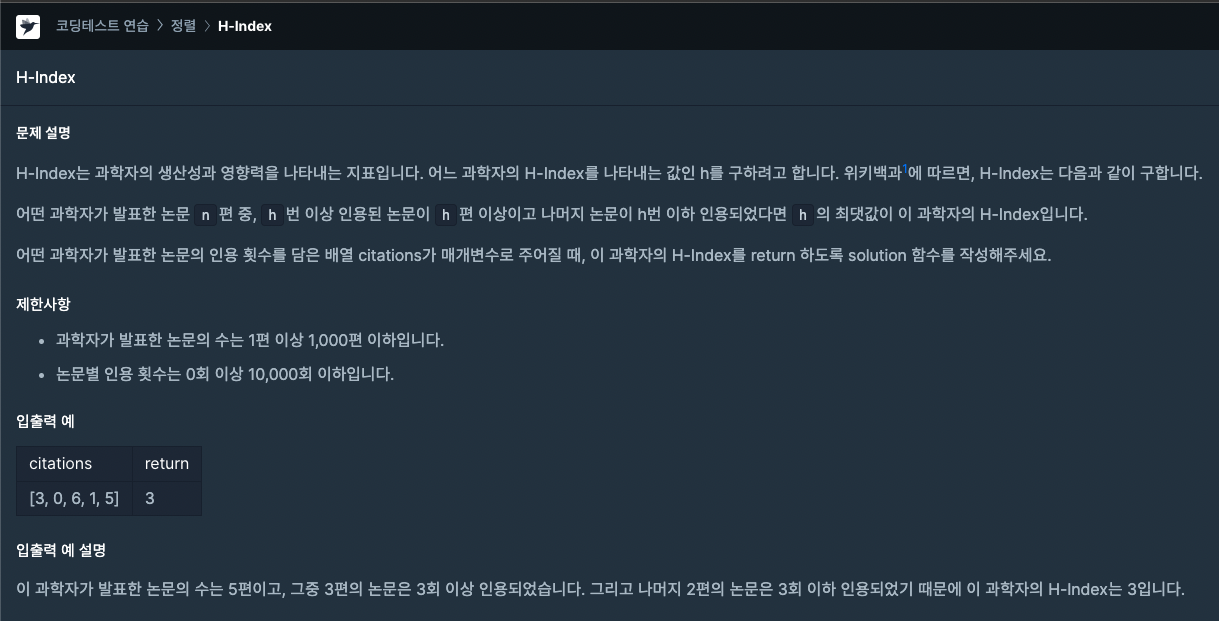
입출력 예시
Input-1
citations = [3, 0, 6, 1, 5]
Output-1
3
문제 풀이
문제 풀이에 앞서 H-index가 뭔지 짚고 넘어가자.
h-index란?
위키피디아에 기재된 내용을 보면 “h-index는 주어진 저자/저널이 각각 최소 h회 인용된 최소 h개의 논문을 출판 한 h의 최대값으로 정의됩니다.” 라고 한다.
위키피디아 내용을 보면 내림차순으로 정렬된 배열이 있어야 h-index를 구할 수 있다.
그리고 각각의 인덱스값과 인덱스에 해당하는 값을 비교해 인덱스가 인덱스에 해당하는 값보다 크거나 같으면 그 값이 h-index라고 이해하면 된다.
말로는 잘 이해가 안되니 citations배열 [3, 0, 6, 1, 5]을 예시로 들어보자.
1
2
3
4
5
6
citations = [6,5,3,1,0] // 내림차순 정렬
i=1, citations=6 -> index<citations -> h-index 아님
i=2, citations=5 -> index<citations -> h-index 아님
i=3, citations=3 -> index==citations -> i값이 h-index
위 과정대로 h-index를 구할 수 있다. 이제 코드를 작성해보자.
1
2
3
4
5
6
Integer[] cit = Arrays.stream(citations).boxed().toArray(Integer[]::new);
Arrays.sort(cit, Collections.reverseOrder());
int i=0;
int answer = 0;
boolean none = false;
먼저 citations를 내림차순 정렬한 cit 배열을 초기화하고, h-index를 담을 answer와 index 값을 담을 i, h가 0으로만 존재할 때를 검증할 none 변수를 초기화했다.
1
2
3
4
5
6
7
8
for(; i<cit.length-1; i++) {
if((i+1)<=cit[i] && (i+2)>cit[i+1]) {
answer = i+1;
break;
}
else if((i+1)>cit[i]) none = true;
}
if(none==false) answer=i+1;
cit에서 마지막-1까지 순회하며 현재 인덱스값이 cit값보다 작거나 같고, 다음 인덱스값이 다음 cit값보다 크면 현재 인덱스값이 h-index임을 표현하였다.
그리고 인덱스 값이 하나라도 크다면 none을 true로 변경한다. none을 통해 인덱스값이 항상 작을 때 마지막 인덱스값이 h-index임을 구할 수 있다.
작성 코드
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
import java.util.*;
class Solution {
public static void main(String[] args) {
int[] citations = {3, 0, 6, 1, 5};
// int[] citations = {10,8,5,4,3};
// int[] citations = {25,8,5,3,3};
// int[] citations = {4,4,4};
// int[] citations = {10,10,10,10,10};
// int[] citations = {0,0,0,0,0};
long start = System.currentTimeMillis();
solution(citations);
long end = System.currentTimeMillis();
System.out.println("\n수행시간 = " + (end-start));
}
public static int solution(int[] citations) {
Integer[] cit = Arrays.stream(citations).boxed().toArray(Integer[]::new);
Arrays.sort(cit, Collections.reverseOrder());
int i=0;
int answer = 0;
boolean none = false;
for(; i<cit.length-1; i++) {
if((i+1)<=cit[i] && (i+2)>cit[i+1]) {
answer = i+1;
break;
}
else if((i+1)>cit[i]) none = true;
}
if(none==false) answer=i+1;
return answer;
}
}
회고
- 문제를 봐도 H-index에 대해 이해가 잘 안되었는데, 링크된 위키피디아 글을 보고 이해하였다.
- 현재 작성한 코드가 맘에 안든다.. 다음에 다시 풀며 간결한 코드로 수정할 예정이다.